Your first MEAN.JS site running on the cloud
Index
- Intro
- Ingredients
- Receipt
- Mongo
- Heroku
- Extra
- References
Intro
In this tutorial I want to change a little bit the subject we've been following during these last months. Today, you will discover how easy can be build an entire site, frontend, backend and finally publish everything on the cloud for FREE and only in a few hours.
By using the MEAN stack and Yeoman we can achieve that in a relatively easy way and once our machine is completely setup repeat this process every time we want. See here below how the full environment looks:
Ingredients
Please, serve yourself with these awesome ingredients so you can cook a very special receipt and impress all your guests:- https://toolbelt.heroku.com/: register in the Heroku site, as this will be our hosting service provider and install its toolbelt application.
- https://nodejs.org/en/: download and install last version.
- https://www.python.org/download/releases/2.7.3/#download: install python v2.7.3 as it's needed to compile native NodeJs addons and it's the current supported version. Make sure that you have a PYTHON environment variable, and it is set to drive:\path\to\python.exe not to a folder.
- https://www.mongodb.org/downloads: this will be our NoSQL database provider. Install it and run the Mongo daemon: mongod.exe, within a console (remember to create c:\data\db folder structure using the default mongo settings).
- Microsoft Build Tools 2013 / 2015
- Finally, within the "Extra" section at the bottom of this post, a stunning windows console called cmder running on your machine. Completely recommended console with some steroids.
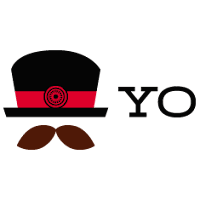
Receipt
Once all the ingredients are installed on your computer, now is the time to start cooking our new application.First of all we need to install globally a few packages in our NodeJS instance by running the following command:
- Yo: Yeoman helps you to kickstart new projects, prescribing best practices and tools to help you stay productive. To do so, they provide a generator ecosystem. A generator is basically a plugin that can be run with the `yo` command to scaffold complete projects or useful parts.
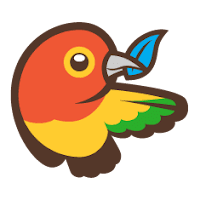
- grunt-cli: this is the build system helping you out to build, preview and test your applications. Gulp is also another popular option for building project.
- bower: this is a package manager used to solve project dependencies. Npm is a common alternative used in the previous command.
- node-gyp: this is a cross platform command line for compiling native ad dons modules for NodeJS https://github.com/nodejs/node-gyp
- generator-angular-fullstack: this is the template for our angular site we'll use with Yo. This particular package comes with Heroku compatibility which will be helpful during the deployment process, you can find more info in its site here.
Finally, everything is ready and we can start cooking by running the following commands:
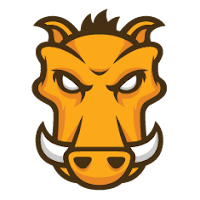
Replace my-new-project with the name of the folder you want, [app-name] with the name of the application you want and have MongoDB running on background. With these three simple commands you end up with a new folder called "my-new-project" and within that folder a complete website created with just one command. Finally compile and run the project locally:
Mongo
For the database hosting I chose mongolab as my provider because is free until 500Mb and really easy to setup. Take a look at their web site and after creating a new account you just need to generate a new Mongo database and copy the URL connection string into your project replacing your localhost Mongo database. Once this is done the last step will be deploy our site on Heroku so it will be open to everybody.
Heroku
For the deployment to Heroku we just need to write some commands within our console and the deployment will be done:
Extra
There is a big component of console commands on this tutorial. For this reason I've tried cmder console for Windows and I think you should also give it a go. It's a really powerful console app which a lot of functionality from Linux systems, besides, it is highly customizable. Follow this tutorial from Andrew Moore to set it up.
References
https://nodejs.org/en
https://toolbelt.heroku.com
http://meanjs.org/generator.html
http://www.awmoore.com/2015/01/14/coding-in-windows-part-1/
https://github.com/yeoman/yeoman